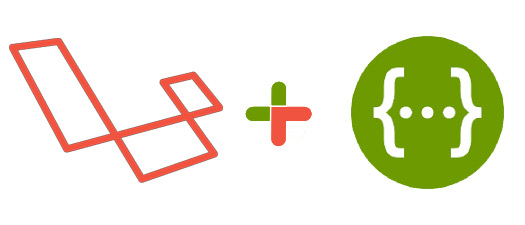
Install Swagger in Laravel
1 step: Create Laravel 5 Project
1 |
composer create-project laravel/laravel l5swagger |
2 step: Install Swagger Package
1 |
composer require darkaonline/l5-swagger |
Or you can install specific verion
1 |
composer require "darkaonline/l5-swagger:5.8.*" |
For use of @SWG annotation we are going to install the following package
1 |
composer require zircote/swagger-php |
Publish config
1 |
php artisan vendor:publish --provider "L5Swagger\L5SwaggerServiceProvider" |
Your composer.json should be like the following
1 2 3 4 5 6 7 8 |
"require": { "php": "^7.1.3", "darkaonline/l5-swagger": "5.8.*", "fideloper/proxy": "^4.0", "laravel/framework": "5.8.*", "laravel/tinker": "^1.0", "zircote/swagger-php": "^2.0" }, |
if any error come then use zircote/swagger-php 2.0 version
1 |
composer require zircote/swagger-php:2.* |
3 Step: Edit .env
Add the following codes in your .env file
1 2 |
L5_SWAGGER_GENERATE_ALWAYS=true SWAGGER_VERSION=2.0 |
4 Step: Create A controller that wants to have Swagger Annotations
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class TestController extends Controller { /** * @SWG\Get( * path="/api/testing/{mytest}", * summary="Get Testing", * operationId="testing", * @SWG\Response(response=200, description="successful operation"), * @SWG\Response(response=406, description="not acceptable"), * @SWG\Response(response=500, description="internal server error"), * @SWG\Parameter( * name="mytest", * in="path", * required=true, * type="string" * ), * ) * */ public function index(Request $request){ echo $request->mytest; } } |
5 Step: Add Annotation in Controller.php (located in \app\Http\Controllers\Controller.php)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
<?php namespace App\Http\Controllers; use Illuminate\Foundation\Bus\DispatchesJobs; use Illuminate\Routing\Controller as BaseController; use Illuminate\Foundation\Validation\ValidatesRequests; use Illuminate\Foundation\Auth\Access\AuthorizesRequests; /** * @SWG\Swagger( * basePath="", * schemes={"http", "https"}, * host=L5_SWAGGER_CONST_HOST, * @SWG\Info( * version="1.0.0", * title="L5 Swagger API", * description="L5 Swagger API description", * @SWG\Contact( * email="[email protected]" * ), * ) * ) */ class Controller extends BaseController { use AuthorizesRequests, DispatchesJobs, ValidatesRequests; } |
Check Swagger Annotations here to learn more about annotations
6: Access our APIs
To access our API navigate to your project with following url
http://localhost/l5swagger/api/documentation
if any error come try to use
1 |
php artisan cache:clear |
1 |
php artisan config:cache |
For authorization
for controller side
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
/** * @SWG\Get( * path="/api/testing/{mytest}", * security={ * {"passport": {}}, * }, * summary="Get Testing", * operationId="testing", * @SWG\Response(response=200, description="successful operation"), * @SWG\Response(response=406, description="not acceptable"), * @SWG\Response(response=500, description="internal server error"), * @SWG\Parameter( * name="mytest", * in="path", * required=true, * type="string" * ), * ) * */ |
and update in l5-swagger.php located in \config folder
1 2 3 4 5 6 7 8 9 |
'security' => [ 'passport' => [ // Unique name of security 'type' => 'oauth2', // The type of the security scheme. Valid values are "basic", "apiKey" or "oauth2". 'description' => 'Laravel passport oauth2 security.', 'flow' => 'password', // The flow used by the OAuth2 security scheme. Valid values are "implicit", "password", "application" or "accessCode". 'tokenUrl' => config('app.url') . '/oauth/token', // The authorization URL to be used for (password/application/accessCode) 'scopes' => [] ], ], |
Congratuation! You learned to document API.
[…] use this documentation https://codefactory.searchmymarket.com/install-swagger-in-laravel/ to create swagger documentation for all APIs in my Laravel […]
[…] use this documentation https://codefactory.searchmymarket.com/install-swagger-in-laravel/ to create swagger documentation for all APIs in my Laravel […]
[…] used this documentation https://codefactory.searchmymarket.com/install-swagger-in-laravel/ to create swagger documentation for all APIs in my Laravel […]